Adding Google Analytics to your Next.js 15 app router is incredibly simple. You don’t need to mess with scripts or long chunks of code. Instead, we’ll use Next.js’s official Google Analytics component and your Google Analytics ID. Let’s get started!
Google Analytics Account Creation
Step 1: Create a Google Analytics Account
Analytics Tools & Solutions for Your Business - Google Analytics
Google Analytics gives you the tools you need to better understand your customers. You can then use those business insights to take action, such as improving your website, creating tailored audience lists, and more.
Google Marketing Platform
- 2.Click the “Get Started Today” button.
- 3.Sign in with your Google account. If you don’t have one, create a new account.
- 4.On the welcome page, click “Start Measuring.”
Step 2: Set Up Your Account
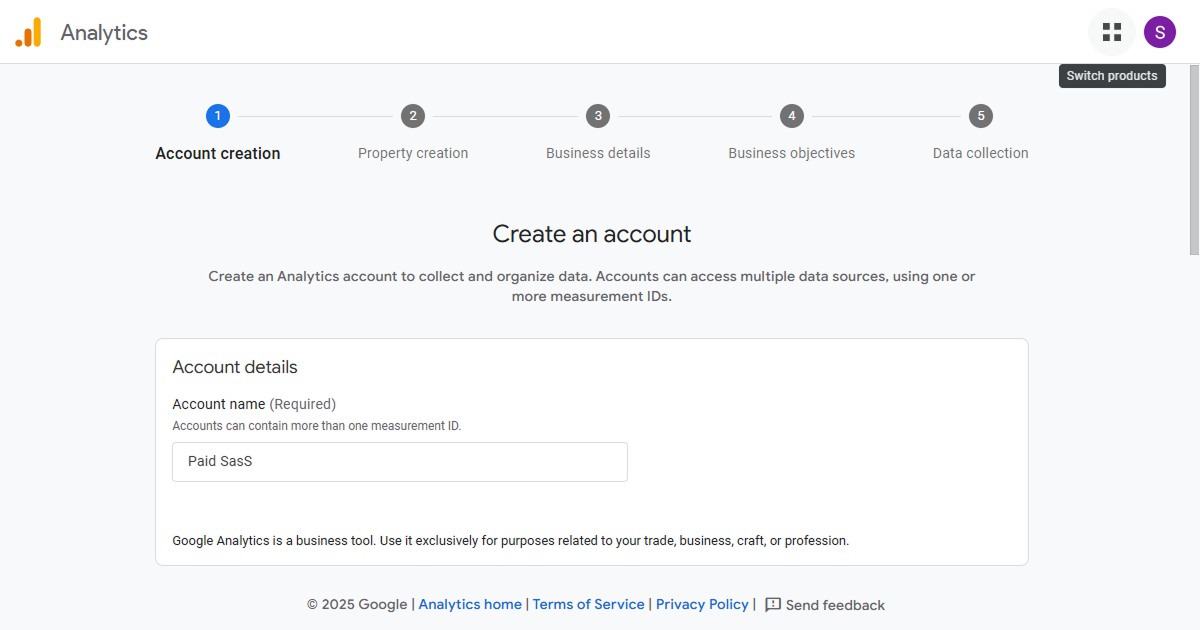
- 1.Fill in the Account Name (e.g., your business or website name).
Note: The account name is different from your Google Analytics ID. You’ll create a property under this account to track your website.
- 2.Scroll down and click Next.
Step 3: Create a Property
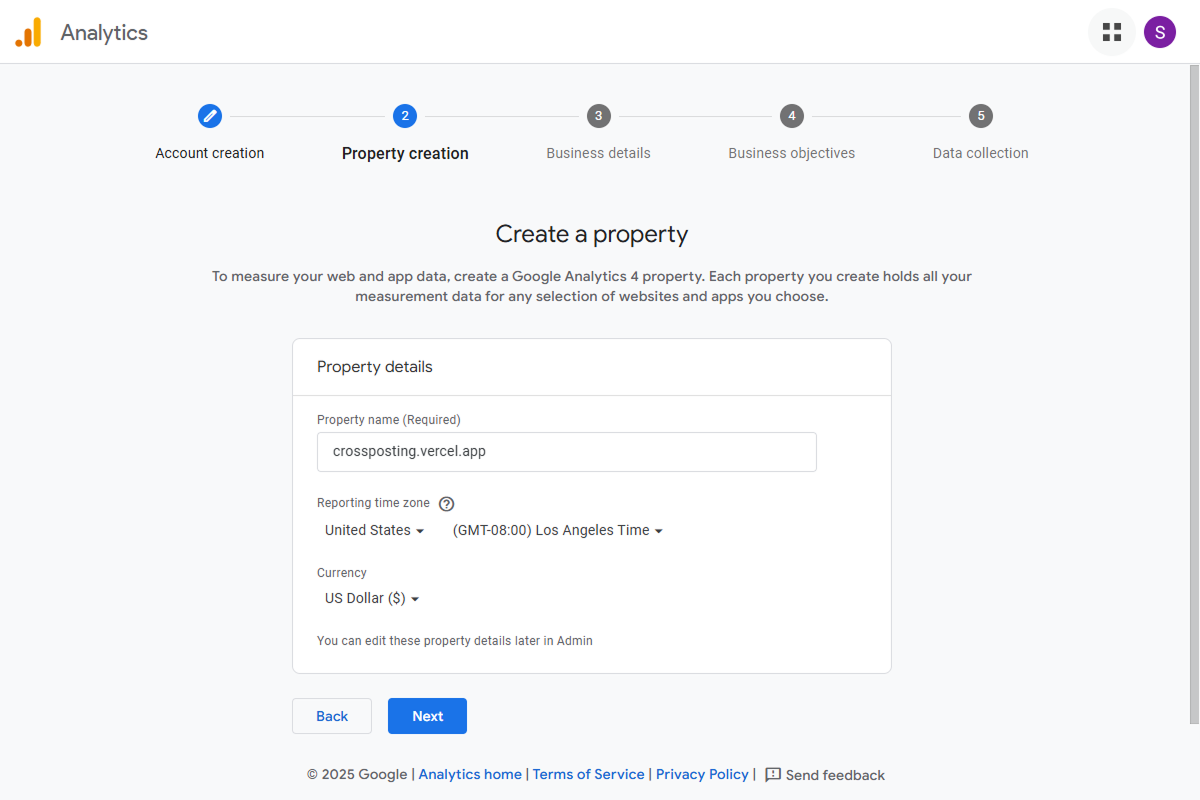
- 1.Enter a Property Name (e.g., your website domain name).
- 2.Adjust the Time Zone and Currency to match your location.
- 3.Click Next.
Step 4: Describe Your Business
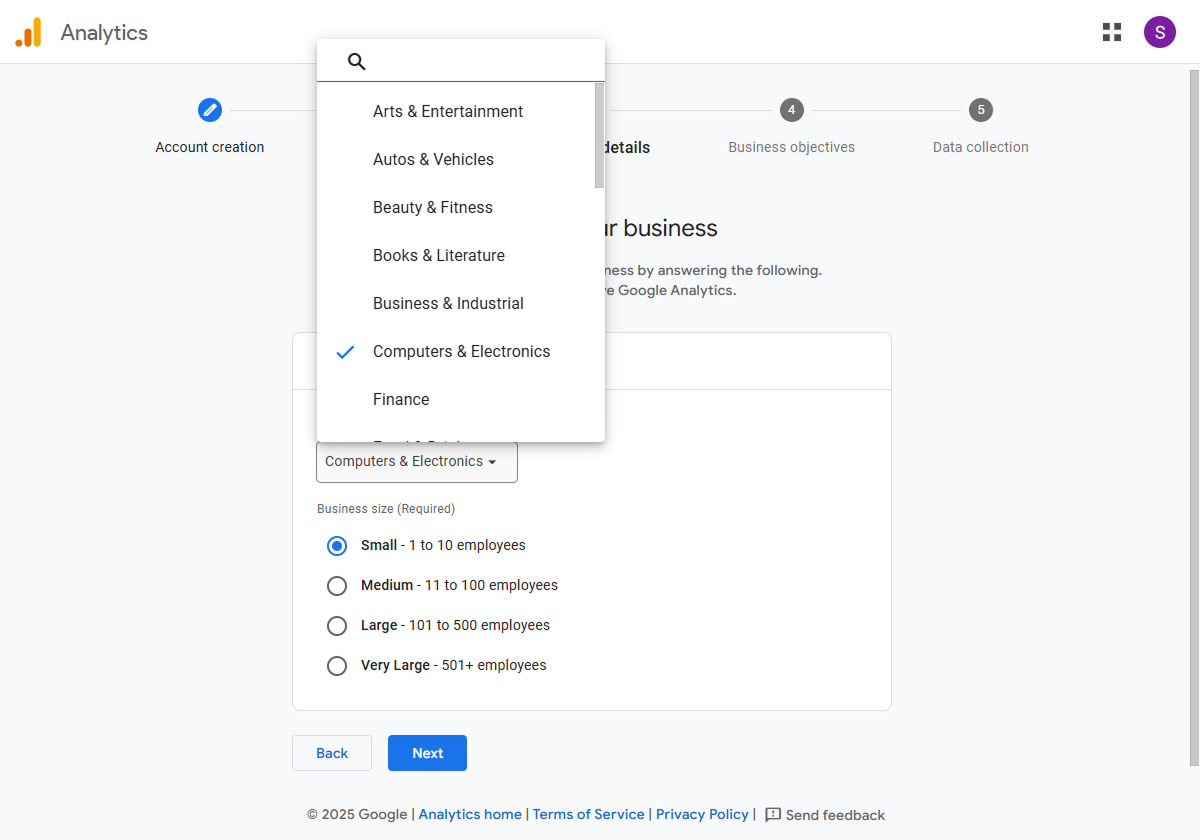
- 1.Select your Industry Category (e.g., Computers & Electronics).
- 2.Choose your Business Size (e.g., Small for 1-10 employees).
- 3.Click Next.
Step 5: Choose Your Business Objectives
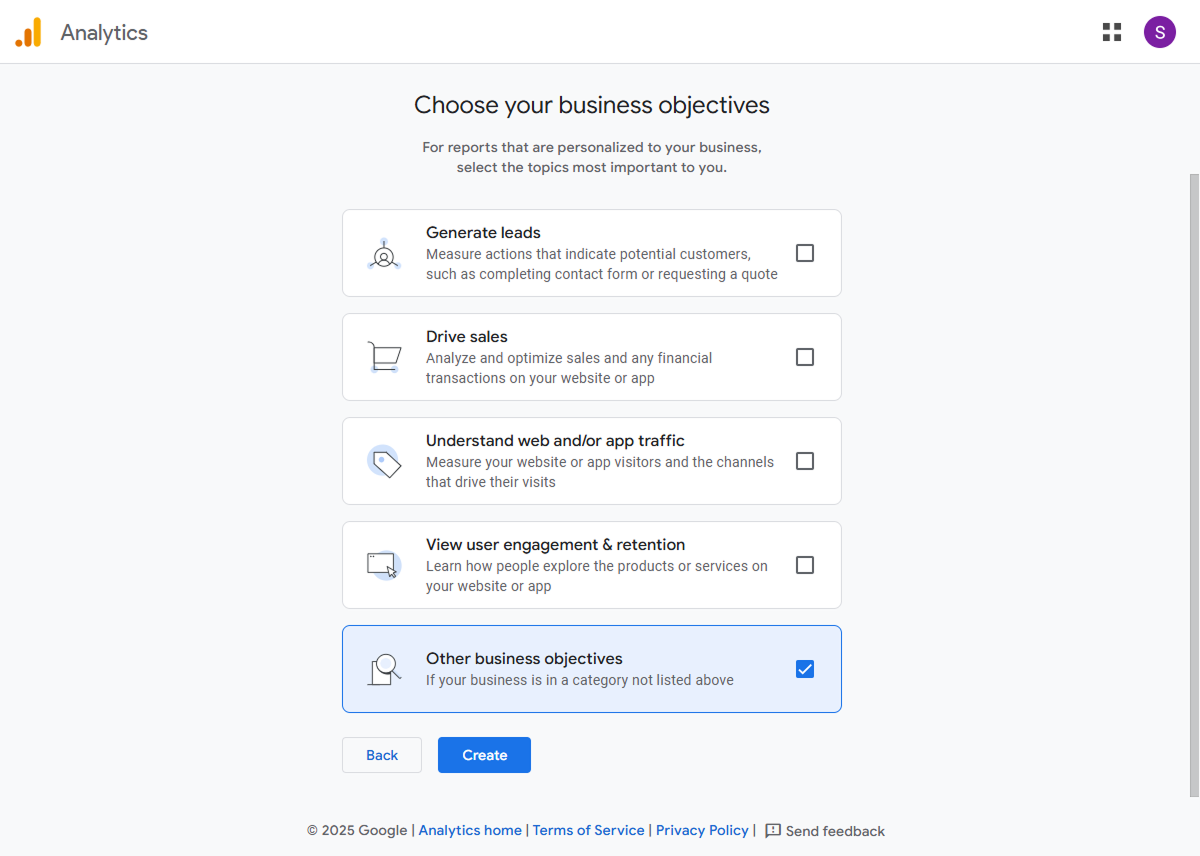
- 1.Select “Other Business Objectives” to enable all features.
- 2.Click Create.
- 3.Accept the Terms of Service and click I Accept.
Step 6: Set Up a Data Stream
- 1.On the “Start Collecting Data” page, select Web.
- 2.Enter your website URL (without
https://
or trailing slashes/
from the end). - 3.Add a Stream Name (e.g., your website name).
- 4.Click Create and Continue.
- 5.Wait for a moment, you will see “Set up google tag” close that page using cross on top.
Step 7: Copy Your Measurement ID
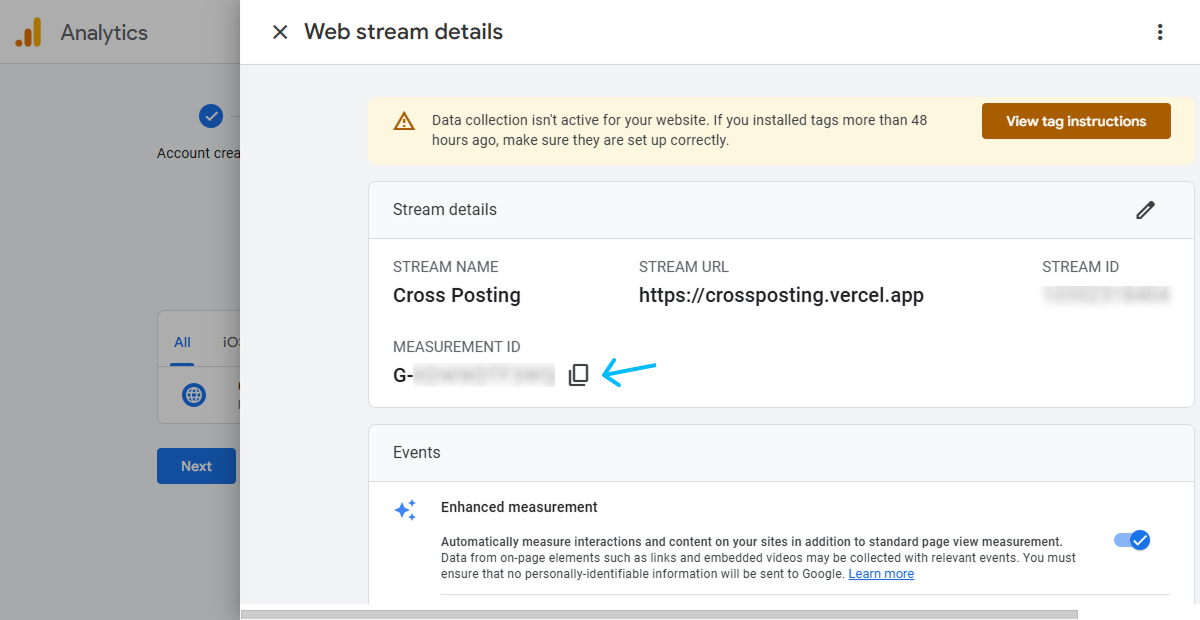
- 1.On the Web Stream Details page, locate your Measurement ID (starts with
G-
). - 2.Copy this ID—you’ll need it for the Next.js setup.
- 3.Close the setup page, Click “Next” & Click “Go to Home” button you will be redirected to Google Analytics dashboard.
Adding Google Analytics to Your Next.js Project
Step 1: Add Google Analytics to Next.js 15
To integrate Google Analytics into your Next.js project, you’ll need to install the official @next/third-parties
library. This library simplifies the process of adding third-party scripts like Google Analytics.
Open your project folder in VS Code and run one of the following commands in the terminal:
For npm
npm install @next/third-parties@latest next@latest
For pnpm
pnpm install @next/third-parties@latest next@latest
For more details read the official documentation
Optimize the performance of third-party libraries in your application with the `@next/third-parties` package.
Step 2: Add Google Analytics to Your Layout
Next, you’ll need to add the Google Analytics component to your app/layout.jsx
or if you are using typescript then app/layout.tsx
For JavaScript use this :
import { GoogleAnalytics } from "@next/third-parties/google";
import "./globals.css";
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>{children}</body>
{process.env.NEXT_PUBLIC_GOOGLE_TAG_MANAGER_ID &&
process.env.NODE_ENV === "production" && (
<GoogleAnalytics gaId={process.env.NEXT_PUBLIC_GOOGLE_ANALYTICS_ID} />
)}
</html>
);
}
For TypeScript use this :
import { GoogleAnalytics } from "@next/third-parties/google";
import "./globals.css";
export default function RootLayout({
children,
}: {
children: React.ReactNode;
}) {
return (
<html lang="en">
<body>{children}</body>
{process.env.NEXT_PUBLIC_GOOGLE_TAG_MANAGER_ID &&
process.env.NODE_ENV === "production" && (
<GoogleAnalytics
gaId={process.env.NEXT_PUBLIC_GOOGLE_ANALYTICS_ID!}
/>
)}
</html>
);
}
Key Points:
- The
GoogleAnalytics
component is only loaded in production mode (process.env.NODE_ENV === "production"
). This ensures no analytics data is sent during development. - The
NEXT_PUBLIC_GOOGLE_ANALYTICS_ID
environment variable stores your Google Analytics Measurement ID.
Step 3: Add Your Measurement ID to Environment Variables
- 1.Create a
.env.local
file in the root of your project (if it doesn’t already exist). - 2.Add the following line to the file:Plain TextCopy
NEXT_PUBLIC_GOOGLE_ANALYTICS_ID=YOUR_MEASUREMENT_ID
Replace
YOUR_MEASUREMENT_ID
with the ID you copied from your Google Analytics account.Why Use Environment Variables?
- Storing your Measurement ID in an environment variable makes it easy to update without modifying your code.
- It also keeps sensitive information out of your codebase.
Step 4: Verify Your Setup
- 1.Deploy Your Next.js Project:
- Make sure to update the environment variable (
NEXT_PUBLIC_GOOGLE_ANALYTICS_ID
) with your Measurement ID before deployment. - Deploy your project to your hosting platform (e.g., Vercel, Netlify, or any other).
- Alternatively, you can test it locally in production mode by runningBashCopy
npm run build
after build is completed run the build using this command
BashCopynpm run start
- 2.Check Real-Time Tracking:
- Visit your website in a new browser tab or incognito window.
- Open your Google Analytics dashboard and navigate to the Real-Time section.
- Confirm that your website traffic is being tracked by checking for active users or pageviews.
- 3.Troubleshooting:
- If no data appears, double-check the following:
- Ensure the
NEXT_PUBLIC_GOOGLE_ANALYTICS_ID
environment variable is correctly set. - Verify that the
GoogleAnalytics
component is only running in production mode (process.env.NODE_ENV === "production"
). - Clear your browser cache or try accessing the site from a different device.
Conclusion
That’s it! You’ve successfully added Google Analytics to your Next.js project using the official @next/third-parties
library. This method is simple, efficient, and ensures your website traffic is tracked without any unnecessary complexity.
Check out my other blogs as well!
How to Add Dark Mode in Next.js 15 App Router with Tailwind CSS V4 (Latest Method)
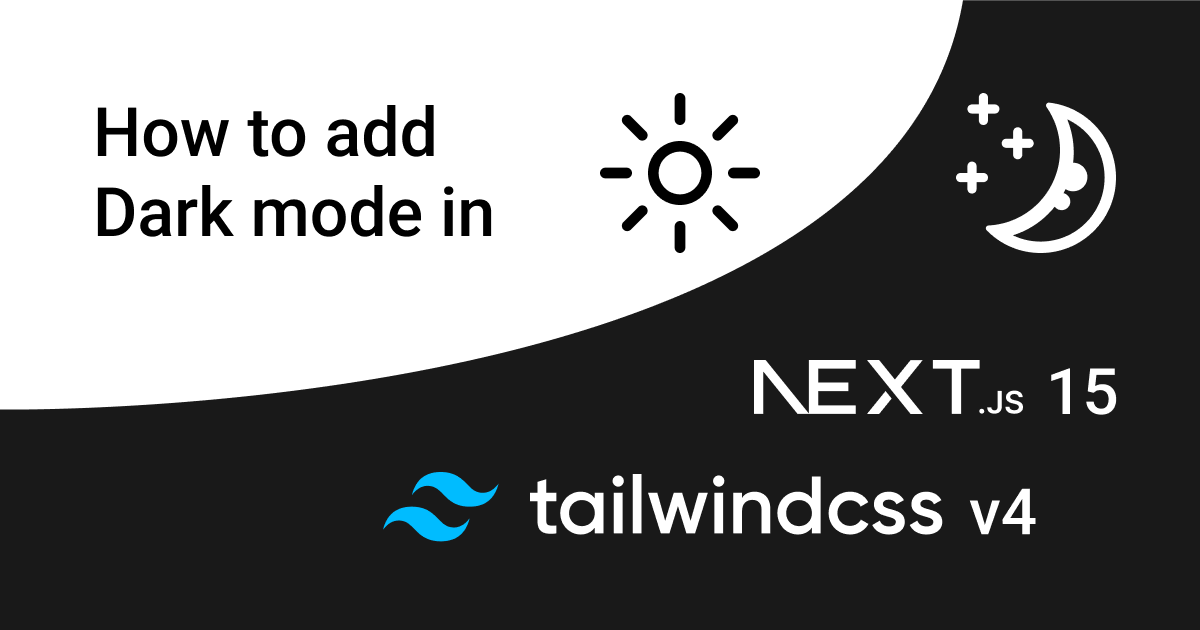
How to Add Dark Mode in Next.js 15 App Router with Tailwind CSS V4 (Latest Method)
Learn how to implement dark and light mode in your Next.js 15 App Router project using Tailwind CSS V4. This step-by-step guide covers the latest changes in Tailwind V4 and Next.js 15 for a seamless theme switcher!
How to Safelist Classes in Tailwind CSS V4
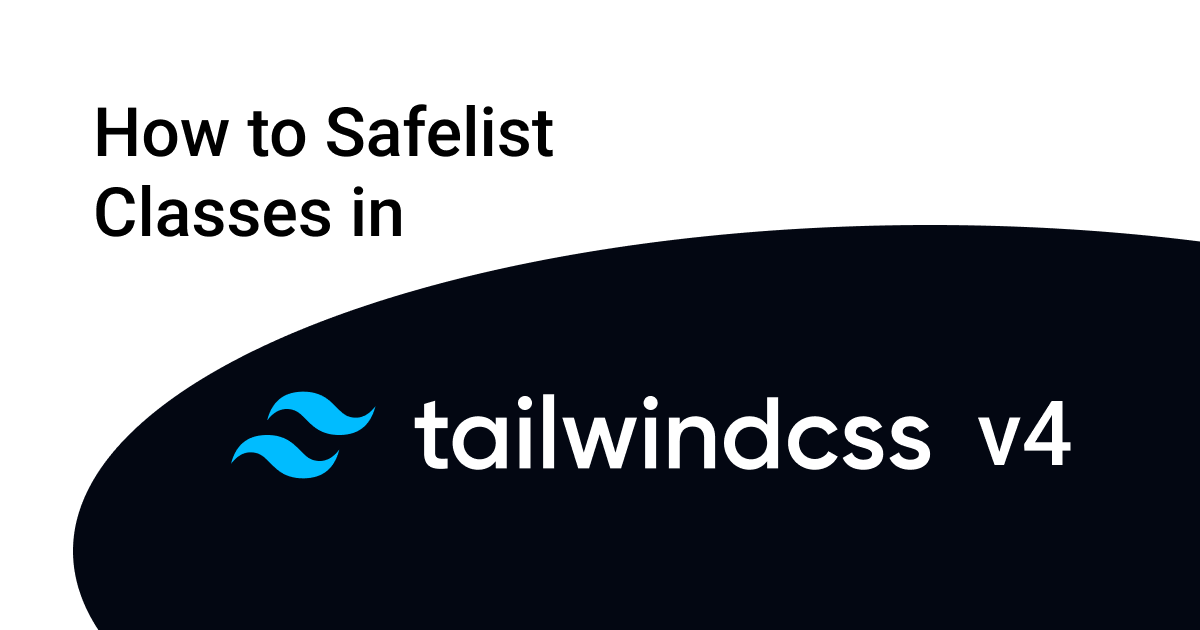
How to Safelist Classes in Tailwind CSS V4
Learn how to safelist classes in Tailwind CSS v4 after the removal of the tailwind.config.js file. Discover the new method to manually define safelisted classes and ensure they are applied correctly in your project